React Lazy Load Image Component - A Must have Library for React Developers
What is Lazy Loading and Why it is important?
Lazy loading is a crucial design pattern in computer programming that delays object initialization until it's necessary. This approach is important for several reasons.
Firstly, it enhances efficiency by reducing initial load time and memory usage, as resources are only loaded as needed. This is particularly beneficial for applications dealing with large volumes of data or content.
Secondly, it improves user experience by providing faster initial page load, which is critical in web development where user engagement can be lost with slow loading times.
Additionally, it can help in reducing resource consumption and costs in cloud-based environments by minimizing unnecessary load on servers.
What is React lazy Load Image Component?
This is a React Component designed to optimize the loading of images and other elements by implementing lazy loading. It includes two key components: LazyLoadImage and LazyLoadComponent. It also features a High Order Component (HOC) - trackWindowScroll - that can be added to any component to track the window scroll position, enhancing performance.
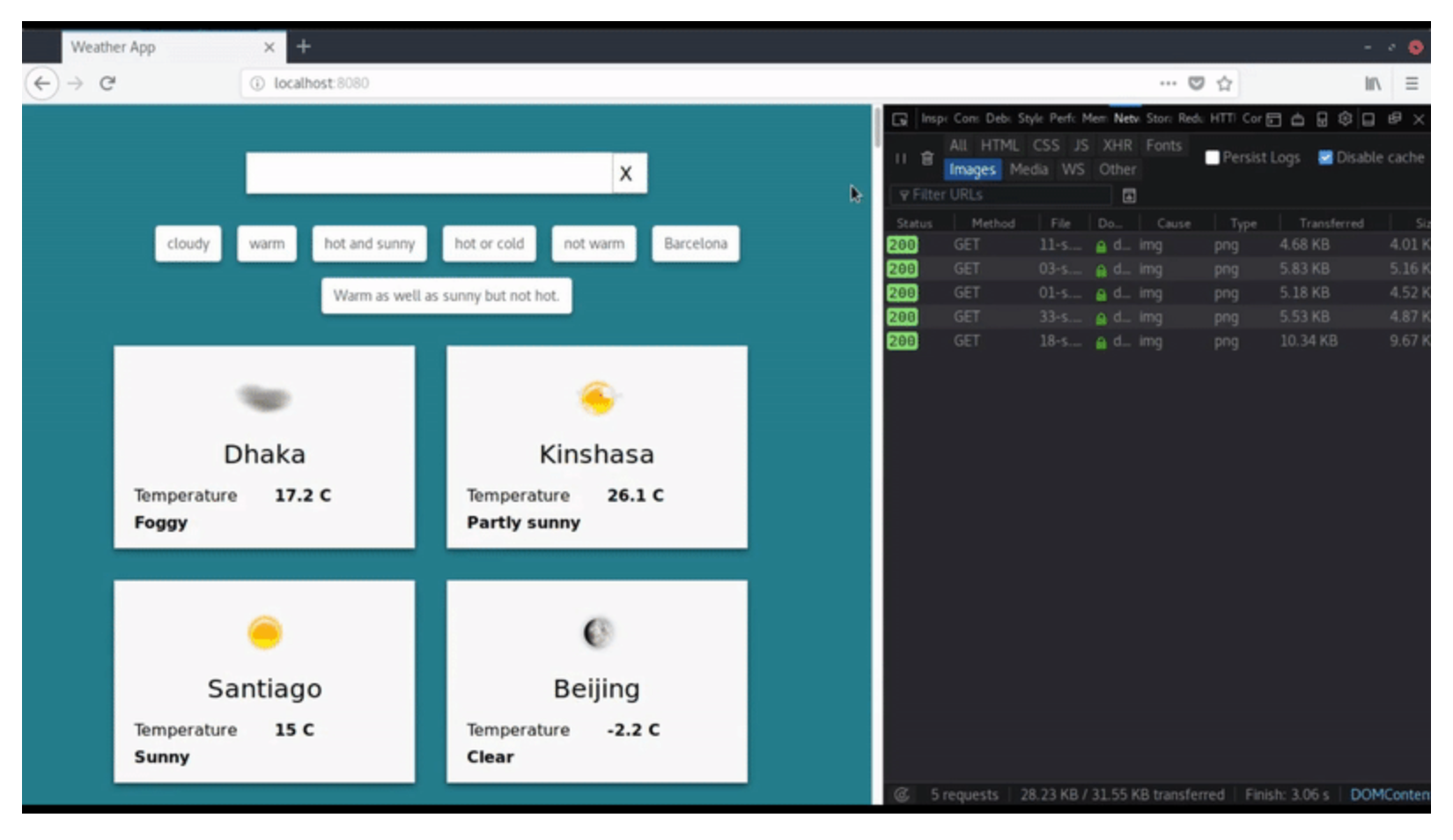
The application efficiently manages scroll events, resize events, and re-renders that may affect the position of the components. It ensures that elements above-the-fold load on initial render. It also provides placeholders with identical sizes to the actual images or components, maintaining layout consistency before the actual element is loaded.
Features
- Includes two components (
LazyLoadImage
andLazyLoadComponent
) and a HOC (trackWindowScroll
) which adds scroll position tracking to any component you wish. - Handles scroll events, resize events and re-renders that might change the position of the components. And, of course, above-the-fold on initial render.
- Placeholder by default with the same size of the image/component.
- A custom placeholder component or image can be specified.
- Built-in on-visible effects (blur, black and white and opacity transitions).
- threshold is set to 100px by default and can be modified.
beforeLoad
andonLoad
events.debounce
andthrottle
included by default and configurable.- Uses IntersectionObserver for browsers that support it.
- Server Side Rendering (SSR) compatible.
- TypeScript declarations hosted on DefinitelyTyped.
Install and use
# Yarn
$ yarn add react-lazy-load-image-component
# NPM
$ npm i --save react-lazy-load-image-component
How to use?
import React from 'react';
import { LazyLoadImage } from 'react-lazy-load-image-component';
const MyImage = ({ image }) => (
<div>
<LazyLoadImage
alt={image.alt}
height={image.height}
src={image.src} // use normal <img> attributes as props
width={image.width} />
<span>{image.caption}</span>
</div>
);
export default MyImage;
Props
LazyLoadImage
Prop | Type | Default | Description |
---|---|---|---|
scrollPosition | Object | Object containing x and y with the curent window scroll position. Required. | |
onLoad | Function | Function called when the image has been loaded. This is the same function as the onLoad of an <img> which contains an event object. | |
afterLoad | Function | Deprecated, use onLoad instead. This prop is only for backward compatibility. | |
beforeLoad | Function | Function called right before the image is rendered. | |
placeholder | ReactClass | <span> | React element to use as a placeholder. |
threshold | Number | 100 | Threshold in pixels. So the image starts loading before it appears in the viewport. |
visibleByDefault | Boolean | false | Whether the image must be visible from the beginning. |
wrapperProps | Object | null | Props that should be passed to the wrapper span when it is rendered (for example, when using placeholderSrc or effect) |
... | Any other image attribute |
Using Effects
LazyLoadImage
includes several effects ready to be used, they are useful to add visual candy to your application, but are completely optional in case you don't need them or want to implement you own effect.
They rely on CSS and the corresponding CSS file must be imported:
import React from 'react';
import { LazyLoadImage } from 'react-lazy-load-image-component';
import 'react-lazy-load-image-component/src/effects/blur.css';
const MyImage = ({ image }) => (
<LazyLoadImage
alt={image.alt}
effect="blur"
wrapperProps={{
// If you need to, you can tweak the effect transition using the wrapper style.
style: {transitionDelay: "1s"},
}}
src={image.src} />
);
License
- MIT license