16 Free React Gallery, Lightbox, and Photo Viewer Libraries
In the ever-evolving world of web development, open-source libraries offer a plethora of opportunities for innovation and efficiency. This guide will take you through some of the finest open-source React libraries for galleries, lightboxes, and photo viewers, providing an invaluable resource for your next project.
These libraries can be used with React-based frameworks such as Next.js or used with the amazing Astro framework.
But before you head into our list, we recommend checking our other related lists that can benefit you as a React developer.
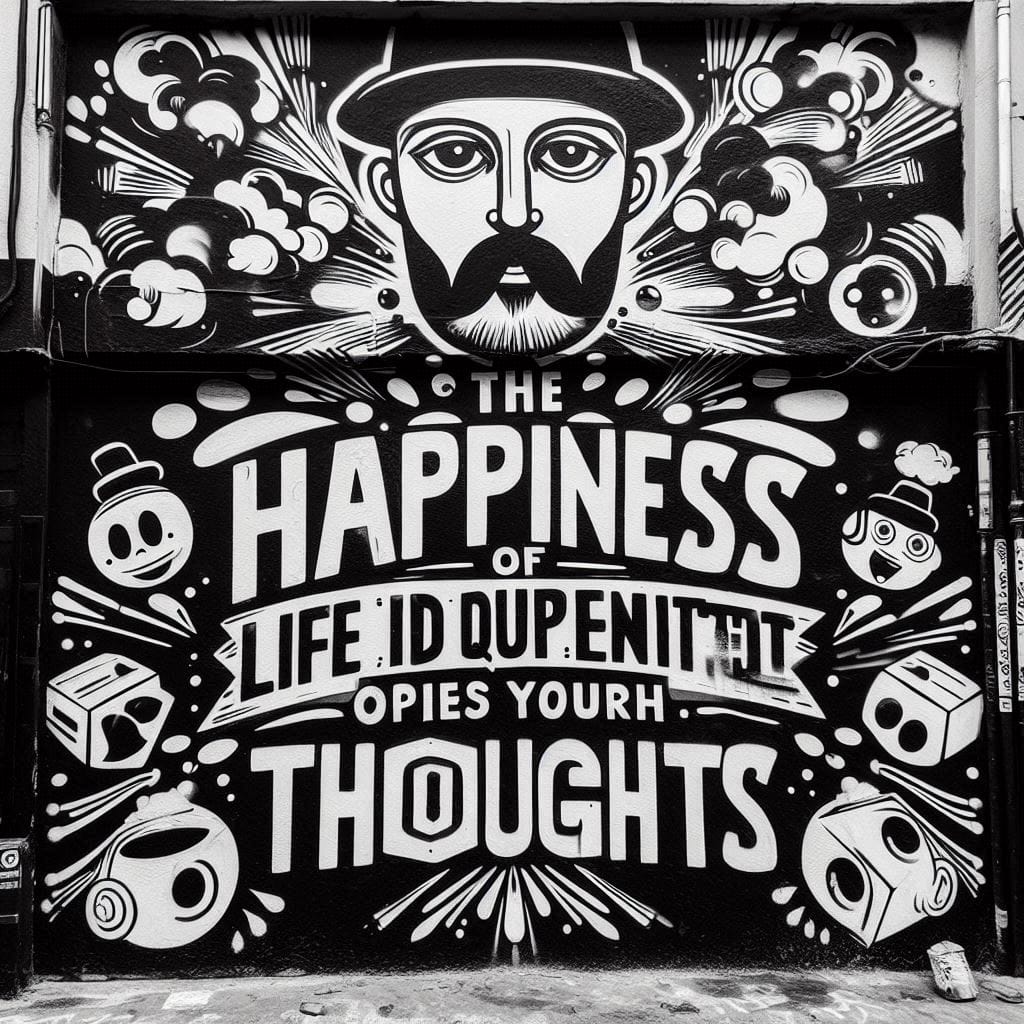
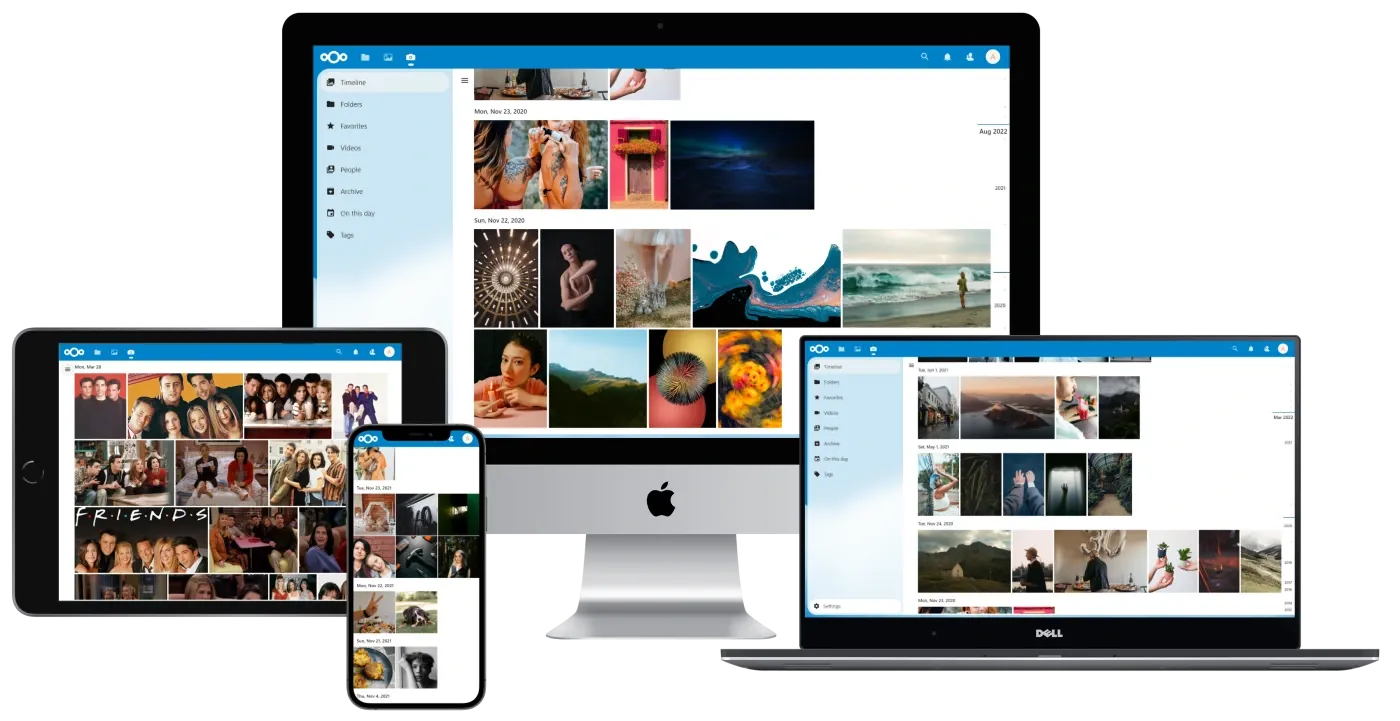
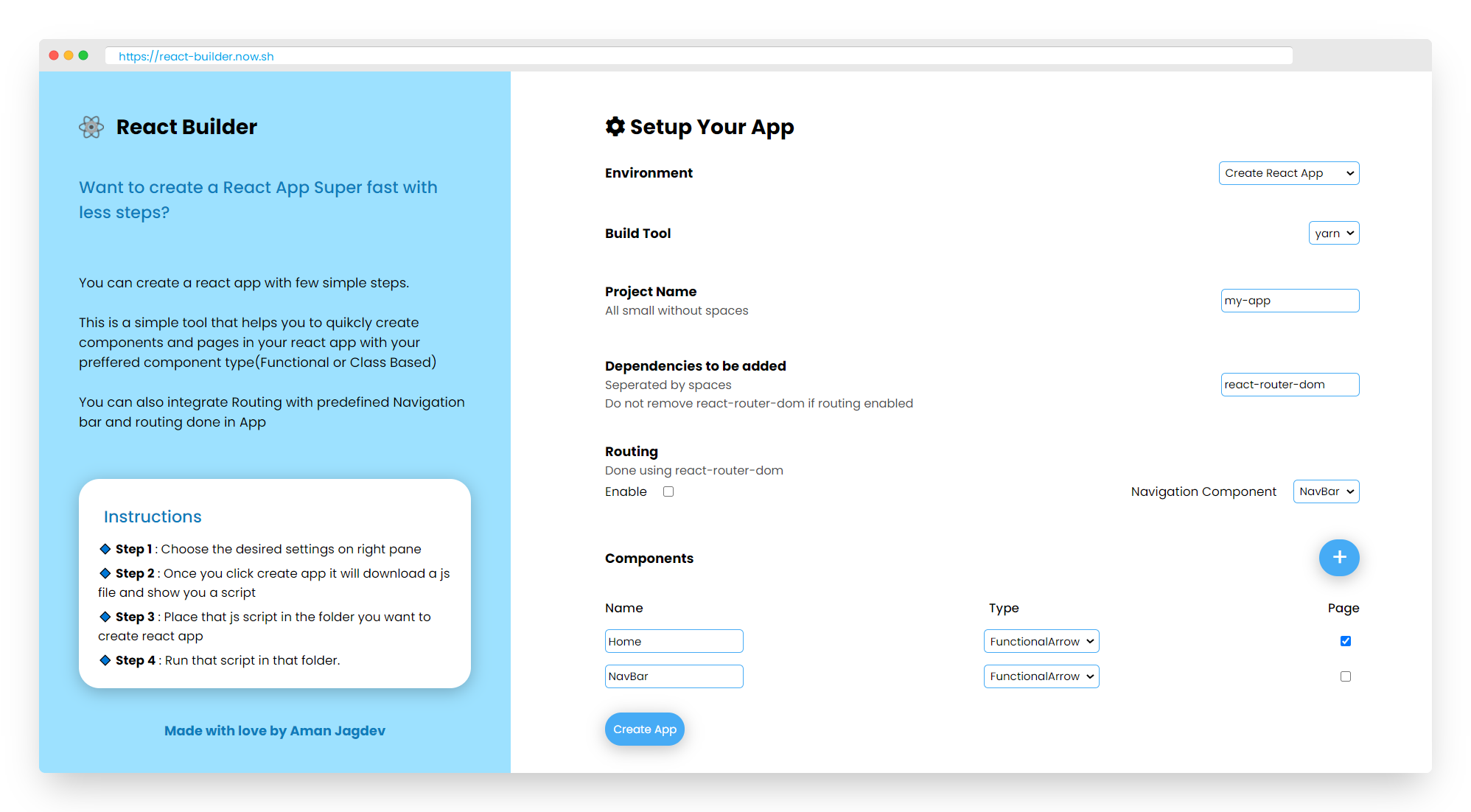
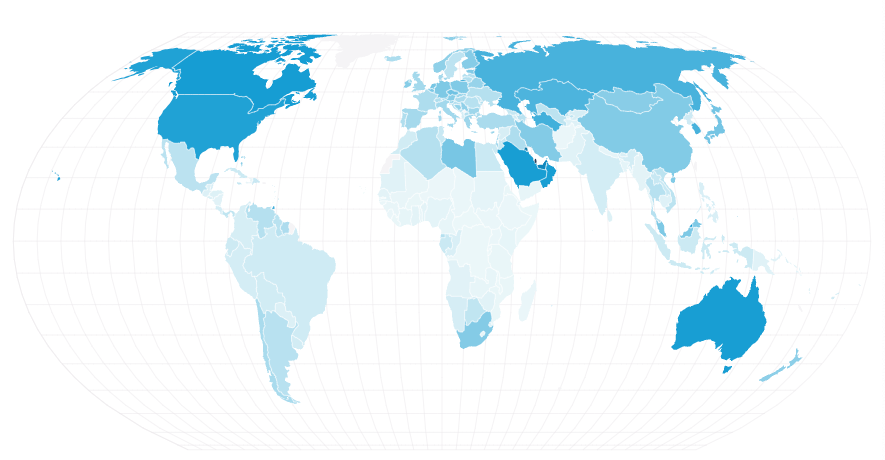
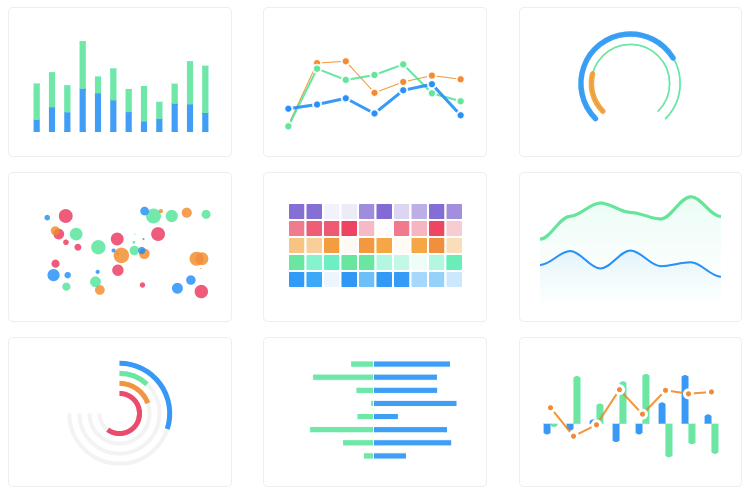
1. React lightbox component
This is an open-source free React library component that enables developers to add a reactive multi-feature Lightbox effect to any React app.
Its current features include zoom, rotation, smooth animation and customizable thumbnails.
The library is an open-source project that is released under the MIT License .
Install
npm install react-lightbox-component
Basic Usage
import Lightbox from 'react-lightbox-component';
const App = () => (
<div>
<Lightbox images={
[
{
src: 'some image url',
title: 'image title',
description: 'image description'
}
]
}/>
</div>
);
2. React Spring Lightbox
React-spring-lightbox is a flexible image gallery lightbox with native-feeling touch gestures and smooth animations.
It replicates the input UX of hardware-accelerated native image applications, supporting gestures and features such as mousewheel, swipe, click+drag, keyboard controls, zooming, panning on zoomed-in images, and spring-based animations. It allows for the implementation of your own UI and requires no external CSS.
It is smooth, lightweight, and comes with highly customizable options.
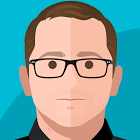
3. Yet Another React Lightbox
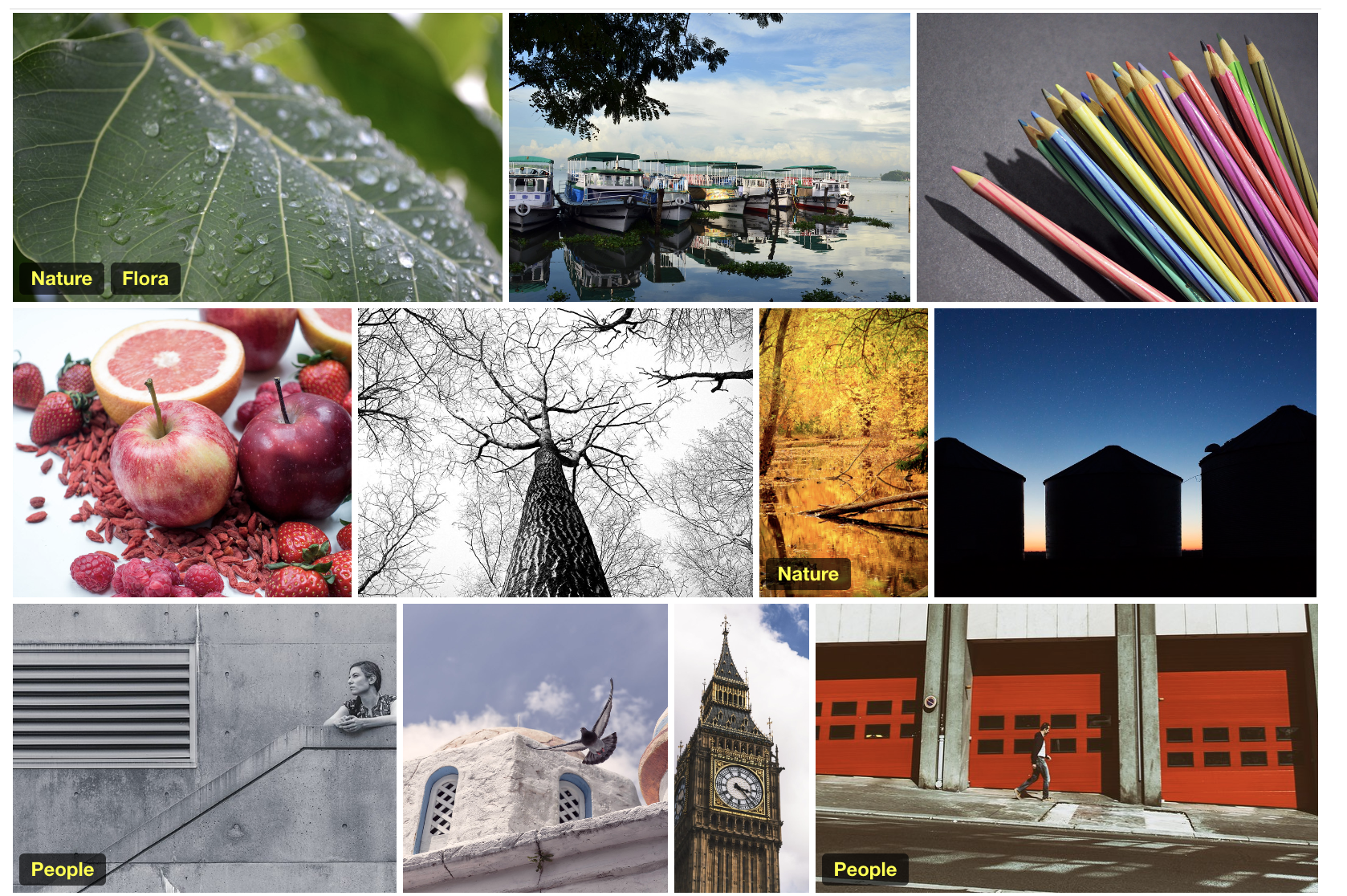
The React Lightbox component is modern, performant, easy to use, customizable, and extendable. It supports React 18, 17, and 16.8.0+, and offers UX support for keyboard, mouse, touchpad, and touchscreen navigation.
It preloads a limited number of images without compromising performance or UX, and never displays partially downloaded images.
You can check the examples here.
Install
npm install yet-another-react-lightbox
Setup
import * as React from "react";
import Lightbox from "yet-another-react-lightbox";
import "yet-another-react-lightbox/styles.css";
export default function App() {
const [open, setOpen] = React.useState(false);
return (
<>
<button type="button" onClick={() => setOpen(true)}>
Open Lightbox
</button>
<Lightbox
open={open}
close={() => setOpen(false)}
slides={[
{ src: "/image1.jpg" },
{ src: "/image2.jpg" },
{ src: "/image3.jpg" },
]}
/>
</>
);
}
4. React LightBox Pack
The React LightBox Pack is a lightweight NPM package built from scratch, specifically for React, with no additional dependencies required. It is customizable and now features added type safety in version 0.2.0.
Install
npm install react-lightbox-pack
or npm i react-lightbox-pack
5. Lightbox-like Image viewer for React
The "Lightbox-like Image viewer for React" is a powerful library that offers a comprehensive and interactive viewing experience. It supports functions such as zoom, rotate, and move, applicable to a single or multiple images. It comes with basic touch support and is capable of responsive design, making it adaptable to various device screens.
Additionally, it supports a full 360-degree rotation, providing a rich user experience. It also includes touch and full keyboard support, demonstrating its versatility across different user interactions.
Install
yarn add react-awesome-lightbox
Setup
import Lightbox from "react-awesome-lightbox";
// You need to import the CSS only once
import "react-awesome-lightbox/build/style.css";
Use
<Lightbox image="image_url" title="Image Title">
6. React Image Lightbox
React Image Lightbox is a flexible component for displaying images in a React project. It features keyboard shortcuts, image zoom, flexible rendering, image preloading, and mobile-friendly capabilities.
Install
npm install react-image-lightbox
Usage
import React, { Component } from 'react';
import Lightbox from 'react-image-lightbox';
import 'react-image-lightbox/style.css'; // This only needs to be imported once in your app
const images = [
'//placekitten.com/1500/500',
'//placekitten.com/4000/3000',
'//placekitten.com/800/1200',
'//placekitten.com/1500/1500',
];
export default class LightboxExample extends Component {
constructor(props) {
super(props);
this.state = {
photoIndex: 0,
isOpen: false,
};
}
render() {
const { photoIndex, isOpen } = this.state;
return (
<div>
<button type="button" onClick={() => this.setState({ isOpen: true })}>
Open Lightbox
</button>
{isOpen && (
<Lightbox
mainSrc={images[photoIndex]}
nextSrc={images[(photoIndex + 1) % images.length]}
prevSrc={images[(photoIndex + images.length - 1) % images.length]}
onCloseRequest={() => this.setState({ isOpen: false })}
onMovePrevRequest={() =>
this.setState({
photoIndex: (photoIndex + images.length - 1) % images.length,
})
}
onMoveNextRequest={() =>
this.setState({
photoIndex: (photoIndex + 1) % images.length,
})
}
/>
)}
</div>
);
}
}
7. Simple-React-Lightbox (SRL)
Simple React Lightbox (SRL) is a tool that allows for the addition of a light-box functionality to a set of images, regardless of whether they are predefined or sourced externally. It was created to address the need for a light-box solution for content that is not known beforehand.
From version 1.3, a gallery with links and images as thumbnails can be created, offering full control for custom galleries.
Install
npm install --save simple-react-lightbox
yarn add simple-react-lightbox
Usage
import React from "react";
import MyComponent from "./components/MyComponent";
import SimpleReactLightbox from "simple-react-lightbox"; // Import Simple React Lightbox
function App() {
return (
<div className="App">
<SimpleReactLightbox>
<MyComponent /> // Your App logic
</SimpleReactLightbox>
</div>
);
}
export default App;
8. The Photo Gallery (Full App -React Photo Gallery)
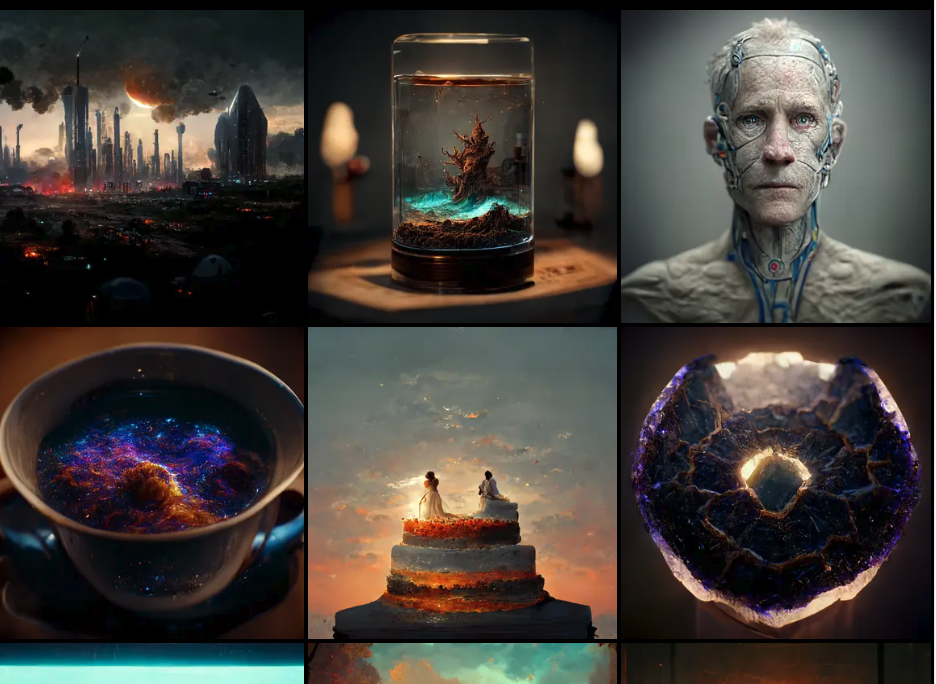
The Photo Gallery is a simple, full-width photo gallery with features such as full-width photo display, lightbox, directory support, automatic image optimization, generation of loading blurs, and automatic dynamic regeneration. It can handle hundreds or thousands of images, supports almost all image file types, and offers image sorting.
Recent updates include support for specific directory regeneration, Imagor support for all file types, and better control over the generated file types.
You can check the demo here.
9. React Image Viewer
This is a simple lightweight React component to display images in a responsive lightbox.
Install
npm install react-image-viewer
Usage
class Demo extends React.Component {
render() {
const style = {
width: 400,
height: 300,
backgroundSize: 'cover'
};
const config = {
viewedImageSize: 0.8,
backgroundOpacity: 0.6
};
return (
<div>
<ImageViewer
style={style}
config={config}
image="http://***"
/>
</div>
);
}
}
10. React Photo View
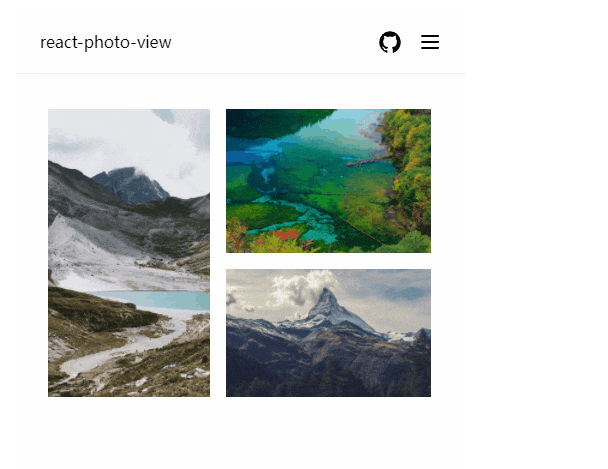
The React photo preview component offers features like touch gestures, drag and pan physical effect sliding, two-finger zooming, animation connection, adaptive image rendering, custom previews, keyboard navigation, custom node expansion, and server-side rendering support. It is based on TypeScript, is 7KB Gzipped, and offers a simple and easy-to-use API.
Install
yarn add react-photo-view
Usage
import { PhotoProvider, PhotoView } from 'react-photo-view';
import 'react-photo-view/dist/react-photo-view.css';
function App() {
return (
<PhotoProvider>
<PhotoView src="/1.jpg">
<img src="/1-thumbnail.jpg" alt="" />
</PhotoView>
</PhotoProvider>
);
}
11. React Simple Image Viewer
React Simple Image Viewer is a component for React. It has a simple image viewer functionality and is available on npm.
Install
$ npm install react-simple-image-viewer
$ yarn add react-simple-image-viewer
How to use
import React, { useState, useCallback } from 'react';
import { render } from 'react-dom';
import ImageViewer from 'react-simple-image-viewer';
function App() {
const [currentImage, setCurrentImage] = useState(0);
const [isViewerOpen, setIsViewerOpen] = useState(false);
const images = [
'http://placeimg.com/1200/800/nature',
'http://placeimg.com/800/1200/nature',
'http://placeimg.com/1920/1080/nature',
'http://placeimg.com/1500/500/nature',
];
const openImageViewer = useCallback((index) => {
setCurrentImage(index);
setIsViewerOpen(true);
}, []);
const closeImageViewer = () => {
setCurrentImage(0);
setIsViewerOpen(false);
};
return (
<div>
{images.map((src, index) => (
<img
src={ src }
onClick={ () => openImageViewer(index) }
width="300"
key={ index }
style={{ margin: '2px' }}
alt=""
/>
))}
{isViewerOpen && (
<ImageViewer
src={ images }
currentIndex={ currentImage }
disableScroll={ false }
closeOnClickOutside={ true }
onClose={ closeImageViewer }
/>
)}
</div>
);
}
render(<App />, document.getElementById('app'));
12. React Images Viewer
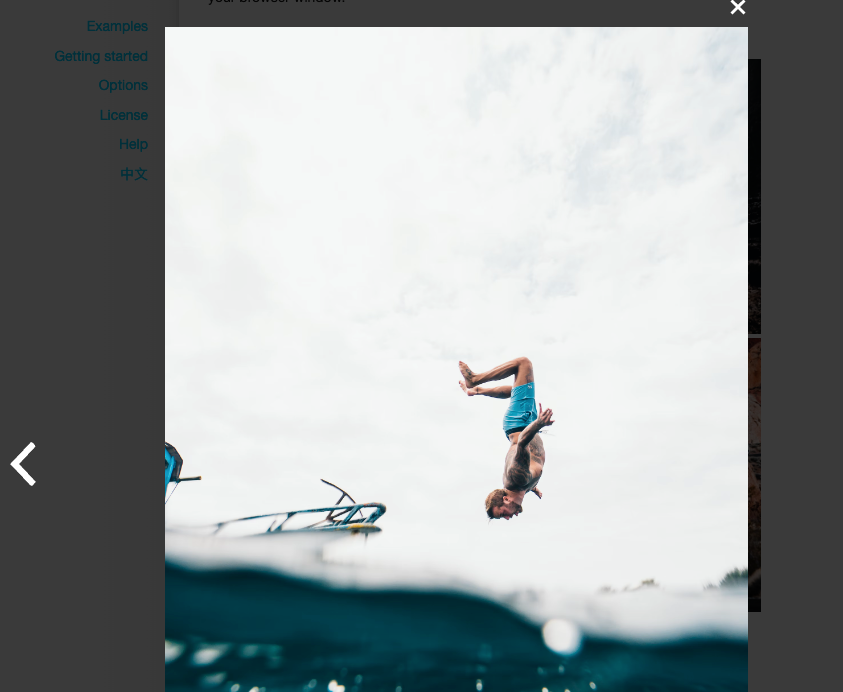
A react library that view photos list easily, and a simple, responsive viewer component for displaying an array of images.
Install
# recommended
yarn add react-images-viewer
# using NPM
npm install react-images-viewer --save
How to use?
import React from "react";
import ImgsViewer from "react-images-viewer";
export default class Demo extends React.Component {
render() {
return (
<ImgsViewer
imgs={[
{ src: "http://example.com/img1.jpg" },
{ src: "http://example.com/img2.png" },
]}
currImg={this.state.currImg}
isOpen={this.state.viewerIsOpen}
onClickPrev={this.gotoPrevious}
onClickNext={this.gotoNext}
onClose={this.closeViewer}
/>
);
}
}
13. React Picture Viewer
React-picture-viewer is a dependency-free picture viewer for React, allowing free dragging and zooming of pictures within the viewport, with adjustable min/max zoom size.
14. React Image Viewer
Yet another free Lightbox and image free component for React.
Install
# ImageViewer requirement
npm install --save react prop-types
npm install --save @mebtte/react-image-viewer
Usage
import React from 'react';
import ImageViewer from '@mebtte/react-image-viewer';
class Demo extends React.Component {
constructor(props) {
super(props);
this.state = {
open: false,
src: 'https://mebtte.com/resource/wallpaper',
};
}
openImageViewer = () => this.setState({ open: true })
closeImageViewer = () => this.setState({ open: false })
render() {
const { open, src } = this.state;
return (
<div>
<button type="button" onClick={this.openImageViewer}>
open
</button>
<ImageViewer
open={open}
src={src}
onClose={this.closeImageViewer}
/>
</div>
);
}
}
export default Demo;
15. Awesome Image Viewer
The Awesome Image Viewer is an open-source free image viewer library that is compatible with React, Angular, Vue, and Typescript and offers features such as lazy loading, zoomability, custom buttons, custom select, swipe on touchscreen, cover size, thumbnail support, navigation with arrow keys, responsive design, dynamic HTML, lightweight, and zero dependency.
16. BaguetteBox.js
This is a simple yet powerful JavaScript library that is built in pure JavaScript and can be easily used with React projects.