How To Backup Docker Volumes Automatically Using Python (Tutorial)
Table of Content
What is Docker, and What is a Docker Volume?
Docker is like a magic box that lets you run applications in isolated environments (called containers). These containers package everything the app needs – like code, libraries, and dependencies – making sure it runs the same way everywhere.
Docker Volumes are how you store persistent data in Docker. Imagine a database running in a container; the database records need to stick around even if the container is destroyed.
Docker volumes are where that data lives.
But what if you lose that volume? Poof! All your data is gone.
That's why you need backups – because data loss is the villain no one wants.
Let’s make sure your Docker volumes are backed up safely with a quick and easy Python script.
Step-by-Step Guide to Back Up Your Docker Volume
Prerequisites
- You should have access to the Docker CLI and the volume you want to back up.
Python installed. Verify with:
python3 --version
Docker should be installed on your system. Check with:
docker --version
Step 1: Create the Python Script
Create a file called backup_docker_volume.py
and paste the following code:
import os
import subprocess
import datetime
# Set your Docker volume name and backup destination
VOLUME_NAME = "your_docker_volume_name"
BACKUP_DIR = "/path/to/backup/folder"
def backup_docker_volume(volume_name, backup_dir):
timestamp = datetime.datetime.now().strftime("%Y-%m-%d_%H-%M-%S")
backup_file = f"{backup_dir}/{volume_name}_backup_{timestamp}.tar"
print(f"Starting backup for volume: {volume_name}")
try:
# Run the Docker command to create a backup archive
subprocess.run([
"docker", "run", "--rm",
"-v", f"{volume_name}:/data",
"-v", f"{backup_dir}:/backup",
"alpine", "tar", "cf", f"/backup/{volume_name}_backup_{timestamp}.tar", "/data"
], check=True)
print(f"Backup successful! Saved to: {backup_file}")
except subprocess.CalledProcessError as e:
print(f"Backup failed: {e}")
if __name__ == "__main__":
if not os.path.exists(BACKUP_DIR):
os.makedirs(BACKUP_DIR)
backup_docker_volume(VOLUME_NAME, BACKUP_DIR)
Step 2: Customize the Script
- Replace
your_docker_volume_name
with your actual Docker volume name. - Set
/path/to/backup/folder
to the directory where you want your backups stored.
Step 3: Install Alpine Image (If Not Already Installed)
This script uses the lightweight Alpine Linux Docker image to create the backup. Pull it with:
docker pull alpine
Step 4: Run the Script
Now, run the script using Python:
python3 backup_docker_volume.py
If everything is set up correctly, you should see:
Starting backup for volume: your_docker_volume_name
Backup successful! Saved to: /path/to/backup/folder/your_docker_volume_name_backup_<timestamp>.tar
Step 5: Automate It with Cron (Optional)
Why not let this run automatically? Add a cron job to back up your volume regularly.
Open the cron editor:
crontab -e
Add a line like this to back up daily at 2 AM:
0 2 * * * /usr/bin/python3 /path/to/backup_docker_volume.py
Final Note
There you go! A simple way to backup your Docker volumes with Python. No data loss nightmares, just peace of mind.
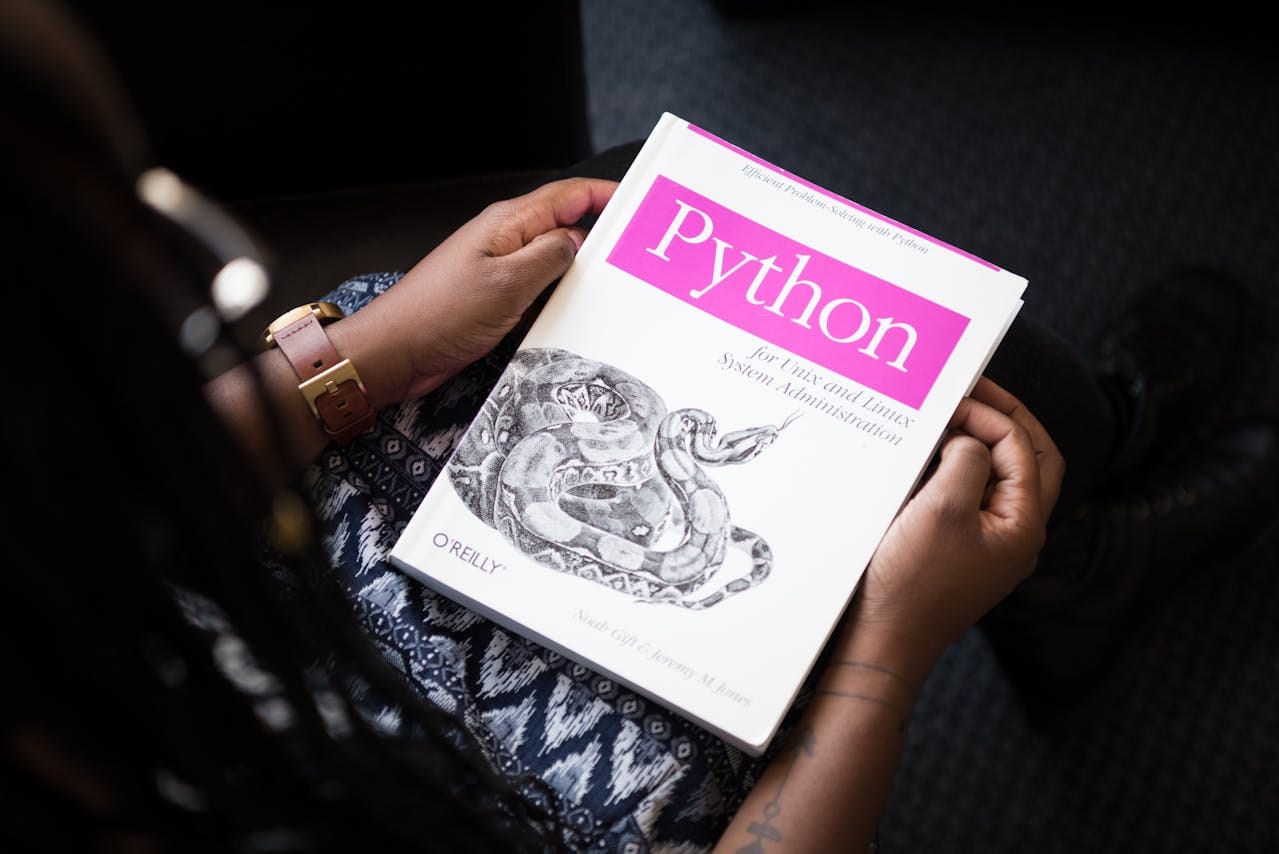
Looking for more Backup Options?
Here you are:
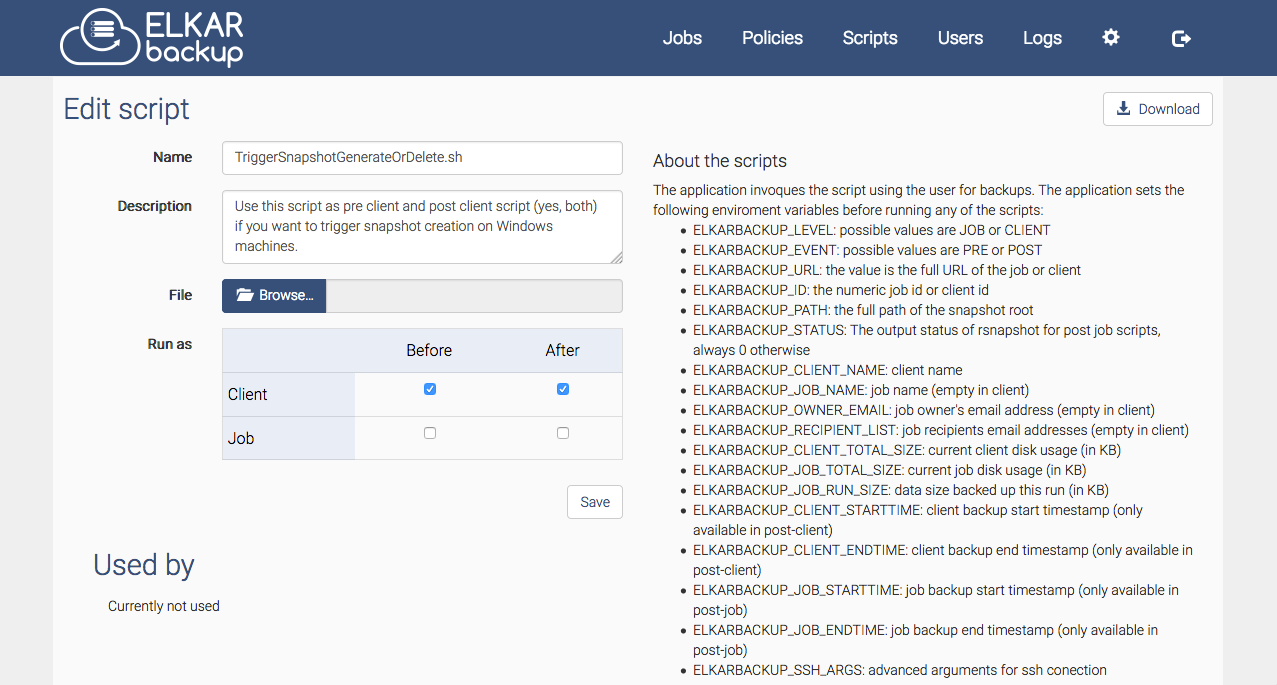
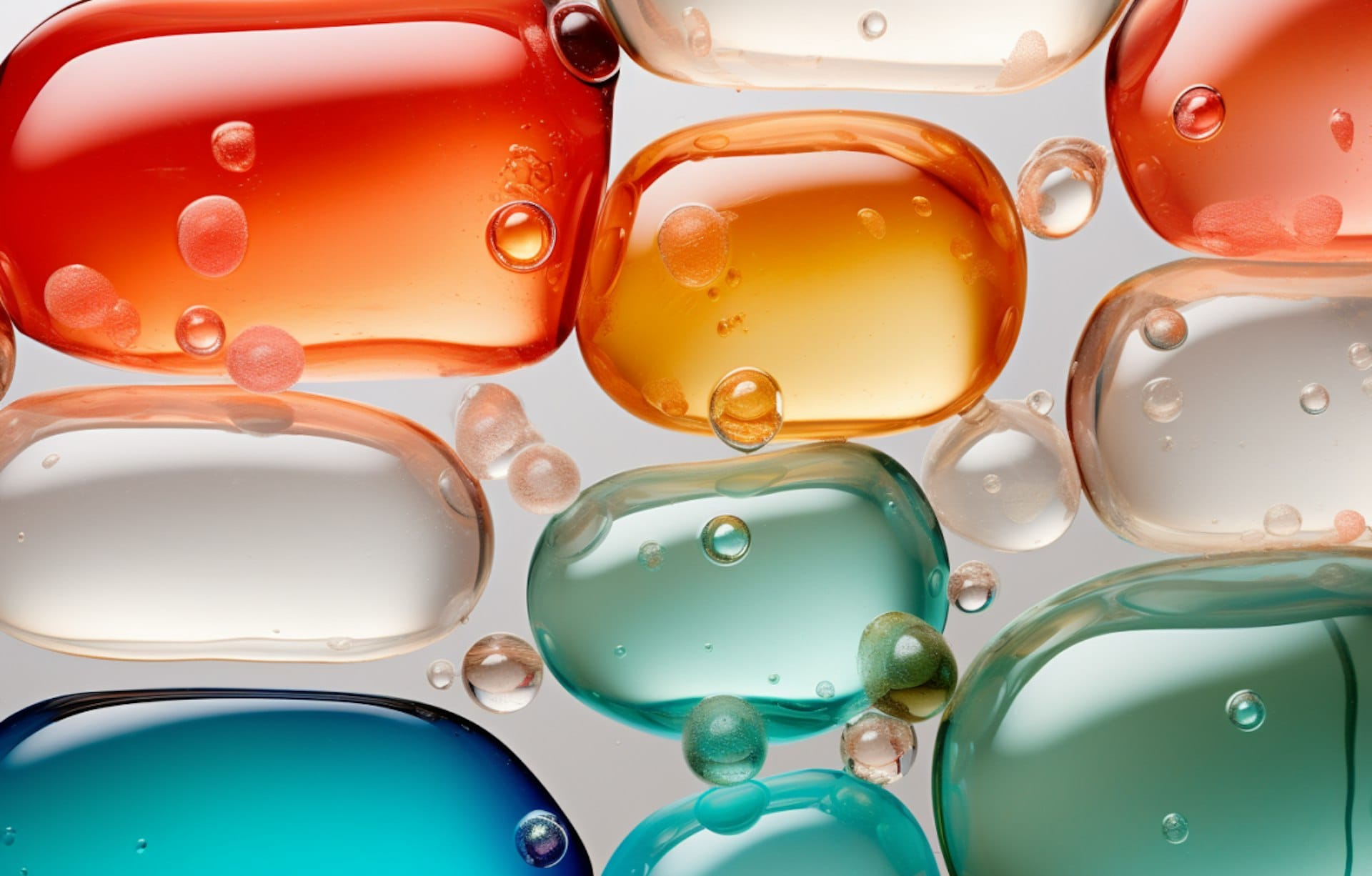